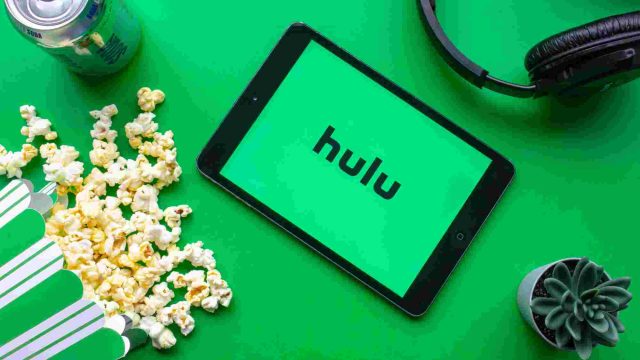
Visuals are powerful online tools, but they can also be performance hogs. If your site relies on bloated image files, visitors may bail before they even see your content. That’s where image transformation steps in – it’s the art of tailoring images for web performance without sacrificing their impact.
Why Image Transformation is a Web Developer’s Best Friend
- The Need for Speed: In an era where milliseconds matter, image transformation is the secret to delivering a fast and seamless user experience. By optimizing images, you accelerate page load times, keeping users engaged and delighted.
- Adapt or Perish: Websites are accessed on a bewildering array of devices with varying screen sizes and resolutions. Image transformation ensures your visuals look stunning on everything from desktops to smartphones.
- Don’t Break the Bank: Large image files eat into bandwidth and storage costs. Optimized images reduce this burden, translating to a healthier bottom line.
- Search Engines Smile: Google and other search engines adore fast websites. Image transformation contributes to better performance, which in turn leads to better rankings and greater visibility for your website.
Mastering the Art of Image Transformation
Image transformation involves a powerful toolkit of techniques:
- Resizing and Cropping: Ensure images are perfectly scaled to fit their containers without distortion or unnecessary white space. This maintains visual coherency across devices.
- Compression: Reduce image file sizes without compromising quality using clever compression algorithms. Think of it like squeezing air out of a balloon – same content, smaller package.
- Format Finesse: Choose the most suitable image format (JPEG, PNG, WebP) based on image type and desired characteristics. Each format has its strengths and weaknesses.
- Adaptive Images: Employ techniques that automatically serve the most appropriately sized image based on the user’s device and connection – the ultimate in flexibility.
- Content Delivery Networks (CDNs): Distribute images across a global network of servers, ensuring lightning-fast delivery to users regardless of location.
Here’s a deeper dive into image transformation techniques, with a few code snippets for illustration:
- Resizing and Cropping:
from PIL import Image
image = Image.open(‘my_image.jpg’)
resized_image = image.resize((400, 300)) # Resize to 400px wide, 300px tall
resized_image.save(‘my_image_resized.jpg’)
Compression:
from PIL import Image
image = Image.open(‘my_image.jpg’)
image.save(‘my_image_compressed.jpg’, quality=80) # Adjust quality (1-100)
Adaptive Images: Use the HTML <picture> element and srcset attribute:
<picture>
<source media=”(max-width: 768px)” srcset=”my_image_small.jpg”>
<source media=”(min-width: 769px)” srcset=”my_image_large.jpg”>
<img src=”my_image.jpg” alt=”Image Description”>
</picture>
Beyond the Basics
Advanced image transformation can open up even more exciting possibilities:
- Lazy Loading: Delay the loading of images below the fold (the initially visible portion of a website) until the user scrolls down, further boosting initial page speed.
Lazy Loading:
const lazyImages = document.querySelectorAll(‘img[data-src]’); // Select images with ‘data-src’ attribute
const lazyLoad = (image) => {
image.src = image.dataset.src;
image.removeAttribute(‘data-src’);
};
// Add an IntersectionObserver to trigger lazyLoad when images enter the viewport
- Image Sprites: Combine multiple smaller images into a single file, reducing the number of server requests a browser has to make.
- Art Direction: Serve different versions of an image tailored to different screen sizes, allowing for more impactful image use on smaller displays.
Tools of the Trade
Image transformation doesn’t have to be a complex, time-consuming endeavor. Specialized services and libraries streamline the process:
- Cloud-Based Solutions: Cloudinary, Filestack, and ImageKit.io offer powerful image transformation capabilities with easy integration into your web development workflow.
- Programming Libraries: Libraries like ImageMagick and Pillow provide granular control if you prefer to handle the transformations within your code.
The Transformation is Worth It
Embracing image transformation might seem like a small detail, but its ripple effects are enormous. By delivering a faster, more responsive, visually stunning web experience, you’ll cultivate happier users, gain an edge in search rankings, and pave the way for the long-term success of your web projects.
Table of Contents